Voice and Chat Integration with HubSpot Using node.js
Integration of a contact center with CRM such as HubSpot is a very common practice to make the customer support or sales work much more efficient. For example, by the associate, each call to a ticket in CRM, makes tracking the call easy to make sure the customer issue is resolved at the end. When the website visitor fills out a contact form, we would like the contact info of the user was saved to the CRM contact table for the future use.
Easiio provides cloud base voice call center and website support and sales center for business. Two types of main entry points into Easiio contact center. One is going through the traditional business phone channel like a traditional call center with multiple levels of IVR supported. The phone call event can trigger the application to write a HubSpot event through a JavaScript program running on node.js. Same for the website chat interface Easiio provide. Moreover, Easiio live chat website plugin is a very powerful combination of several important tools for customer service and sales. It has an automatic chat response called ITR (Interactive Chat Response) with many powerful features built-in.
Easiio call center features:
- Call queue
- Programable multi-level IVR
- IVR call branch to external program running on Node.js
- Call reports of the whole team.
- CRM integration, SalesForce and HubSpot
- Call script
- Call notes
- Outbound call center features. Power dialer.
- Light weight CRM
Easiio website live chat plugin features:
- Programable multi-level ITR
- Customizable promotion item
- Customizable form item for user to fill out
- Display picture, video and files
- Video call
- Desktop share call
- Website visitor location, page visit, browsing time
- Website visitor statistics
- Support chat reports.
- Customizable chat distribution algorithm, by location, by page visit, by topic, by skill level.
- Total website visitor conversion tools.
With the powerful features of the Easiio call center/business phone and website customer contact center features, how can we extend the functionality and integrate with the very powerful HubSpot CRM and marketing services. We introduce the integration of both IVR and ITR in two section
Easiio Call Event to HubSpot sample
When there is a phone call incoming into the Easiio call center, it can be configured to send the event to the external HTTP API. The configuration screen as shown in the figure using Advanced Configuration->Event Notification menu.

Once the event configured to be sent to the HTTP API. When call come in, there event with the details will be sent to the server. In our example, we use the JavaScript running on node.js to process the event and save it as ticket to HubSpot.
Here is the sample code to handle the event send from Easiio Business Phone System and processed with JavaScript on node.js
if(request.method === "POST") {
if (request.url === "/call_event_general") {
var requestBody = '';
const queryObject = url.parse(request.url,true).query;
console.log(queryObject);
console.log("post call_event_answer 1.0");
request.on('data', function(data) {
requestBody += data;
if(requestBody.length > 1e7) {
response.writeHead(413, 'Request Entity Too Large', {'Content-Type': 'text/html'});
response.end('<!doctype html><html><head><title>413</title></head><body>413: Request Entity Too Large</body></html>');
}
});
request.on('end', function() {
console.log("post end", requestBody);
var formData = JSON.parse( requestBody );
var request = require("request");
// on event ring create a ticket at hubspot
if (formData.event === "event_ring") {
var options = {
method: 'POST',
url: 'https://api.hubapi.com/crm/v3/objects/tickets',
qs: {hapikey: apikey},
headers: {accept: 'application/json', 'content-type': 'application/json'},
body: {
properties: {
property_number: '17',
property_dropdown: 'choice_b',
property_radio: 'option_1',
property_string: formData.caller + ' called ' + formData.called,
property_multiple_checkboxes: 'chocolate;strawberry',
property_checkbox: 'false',
property_date: '1572480000000'
}
},
json: true
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
}
// Add code here to handling these call info. It maybe a API call to other applications.
console.log("post end 1.0", formData.caller , " ", formData.called, " ", formData.event);
response.write('[]');
response.end('');
});
}
}
Basically, the sample code parses the event data in the format of JSON, and get several parameters from the calls such as called number and caller number. Using HubSpot for nodejs API to post the parameters from the call event to create a ticket with the caller and called phone number as one of the ticket parameters.
Easiio website live chat with HubSpot
Easiio website chat plugin has the feature of an embed from inside the chat windows. Easiio admin can create as many forms as needed and could be a very complex form to run inside the live chat window. With ITR and form, a developer can collect information from customers and submitted to third party applications such as HubSpot and SalesForce, or their own applications.
Here is the image of the Contact form
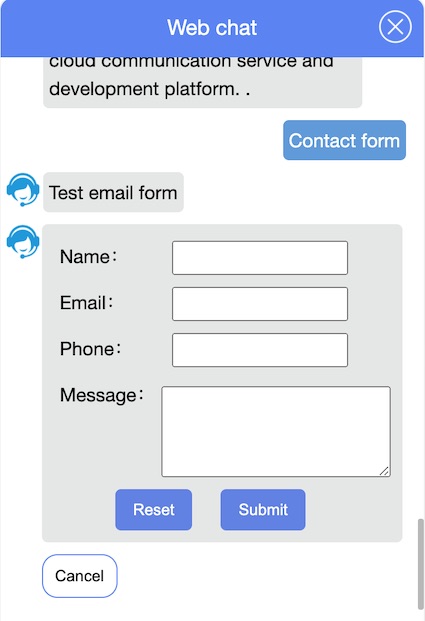
Here is the image of the Call me form
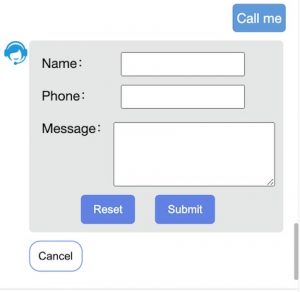
When a user on website chat submits a contact form, the ITR configuration can send the form data to an external for example a JavaScript running on Node.js. The script can then talk to HubSpot to record the new contact. Here is the configuration of the ITR setting the form handling API to a specific URL (Data Url field).

Here is the sample code to write to the HubSpot with JavaScript on node.js.
if(request.method === "POST") {
if (request.url === "/contact_form_submition") {
var requestBody = '';
console.log("post contact_form_submition");
request.on('data', function(data) {
requestBody += data;
if(requestBody.length > 1e7) {
response.writeHead(413, 'Request Entity Too Large', {'Content-Type': 'text/html'});
response.end('<!doctype html><html><head><title>413</title></head><body>413: Request Entity Too Large</body></html>');
}
});
request.on('end', function() {
console.log("post end", requestBody);
var formData = JSON.parse( requestBody );
console.log("post contact_form_submition end 1.0", formData.name , " ", formData.email, " ", formData.phone, " ", formData.message);
var request = require("request");
var name = formData.name.split(/[ ,]+/);
var options = { method: 'POST',
url: 'https://api.hubapi.com/contacts/v1/contact/',
qs: { hapikey: apikey},
headers:
{
'Content-Type': 'application/json' },
body:
{ properties:
[ { property: 'email', value: formData.email },
{ property: 'firstname', value: name[0] },
{ property: 'lastname', value: name[1] },
{ property: 'website', value: '' },
{ property: 'company', value: '' },
{ property: 'phone', value: formData.phone },
{ property: 'address', value: '' },
{ property: 'city', value: '' },
{ property: 'state', value: '' },
{ property: 'zip', value: '' } ] },
json: true };
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
//var responseBody = [ { "id": 1, "action": "play", "text": "submit your form. name: "" voice mails are to your pre-configure cell phone by SMS." }]';
response.write('[ { "id": 1, "action": "play", "text": "submit your form. We will contact you soon." }]');
response.end('');
});
}
}
This sample code handles the form submitted by website visitor using Easiio live chat window. The form data send to the script using JSON format. We just need to parse the JSON format and get the email, first name, last name, phone number, and comments. Save it to HubSpot Contact object.
Conclusion of HubSpot Easiio API integration
Using Easiio call notification event or form submission event and handle inside a nodejs program is a great way of doing third-party integration such as integrate with HubSpot. With this simple and easy to use features of Easiio call center and website contact center, any call or live chat can be integrated to CRM or any third party application. Register to start our free trial and see how these can simplify many third party integration for your business and project. You can download the complete source code at the Github of this sample. The sample code showing here is inside JavaScript file hubspot.js.
See how to sign up for Website chatbot. Easiio Web Chat Plugin Guide