Easiio webchat integration with Microsoft QnAMaker using Node.js
Chatbots are a revolution in customer communication. Nowadays, companies are relying heavily on chatbots to automate and reduce the workload on humans. According to reports, over 80 percent of businesses are considering implement chatbots on their website in the near future.
Many great bot frameworks available such as Amazon Lex, Microsoft Azure AI LUIS, Google DialogFlow, IBM Watson, etc. In this blog, we will show how to use these BOT framework to build a website chatbot with Easiio using Microsoft QnAMaker as an example.
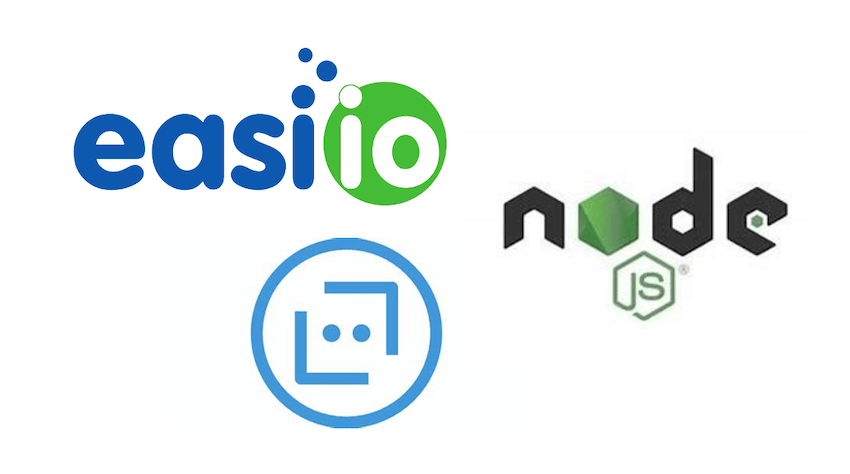
Microsoft QnAMaker
Microsoft QnA Maker is a cloud-based Natural Language Processing (NLP) service that allows you to create a natural conversational layer over your data. It is used to find the most appropriate answer for any input from your custom knowledge base (KB) of information. It is part of the Microsoft Azure AI technology.
Microsoft QnA Maker is commonly used to build conversational client applications, which include social media applications, chat bots, and speech-enabled desktop applications.
When to use Microsoft QnA Maker:
- static information
- provide the same answer to a request, question, or command
- filter static information based on meta-information
- manage a bot conversation that includes static information
Easiio Webchat and Chatbot Integration with QnAMaker
Easiio provides a cloud call center and website chatbot and live-chat service. For the website chatbot and live chat interface. It provides a very flexible application framework for integration with any third-party application using API. For example, it can integrate with CRM, customer support software, and practically any service that provides API access, including the chatbot API such as Amazon Lex as in the previous blog and Microsoft QnAMaker.
Now, let's get started with the implementation of the Microsoft QnAMaker bot with Easiio web chat.
First, we will create Microsoft QnAMaker instance and then expose the functionality using API. Second, we will build a node.js module to handle the request from Easiio webchat framework and Microsoft QnAMaker API return value. Third, we will configure the Easiio webchat to use the Microsoft QnAMaker.
Creat and test QnAMaker instance
Follow Microsoft document "Create a new QnA Maker service" to create a QnAMaker instance. I created an instance called easiioqna. After creating the service instance, you can start the configuration of the QnAMaker details. It supports importing QnA from a website URL if you already have a web page for QnA.
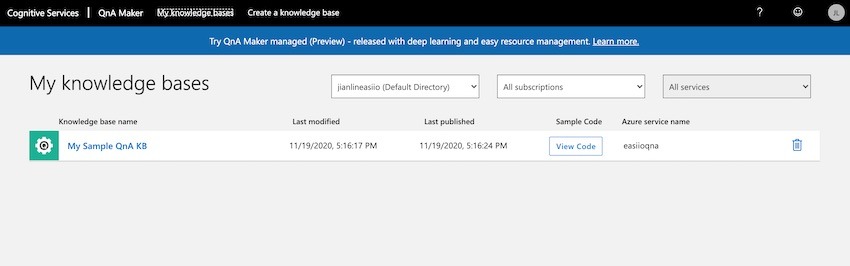
You can enter Questions and Answers for your QnA. For each question, you can enter multiple alternatives for better match of your questions.
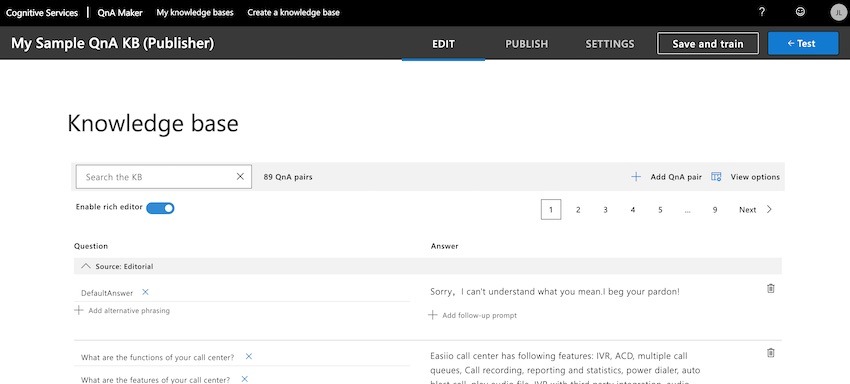
After enter the list of your QnA into the systems, you can now test it by first click "Save and train" and then click on the "+Test" button on the right hand corner of the console. Now, you enter the question on the text field and then hit enter, you should see how your QnAMaker response to your question.
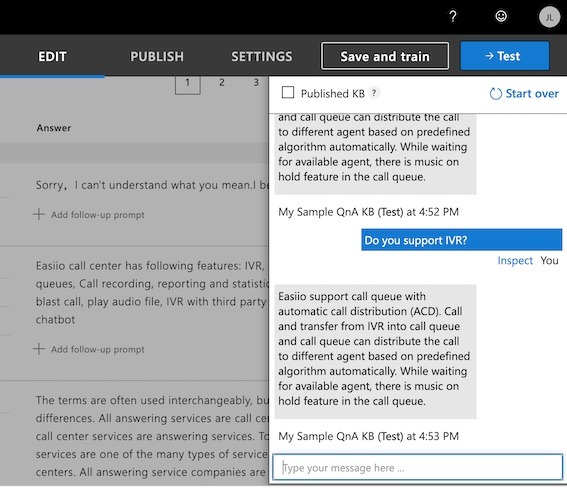
After we test the QnAMaker inside the console and happy with it. The next steps is to test it outside the console using the command line tool curl.
From the QnAMaker console "My knowledge bases" tab, click on the "View code". You can copy the curl command and test it from command line.
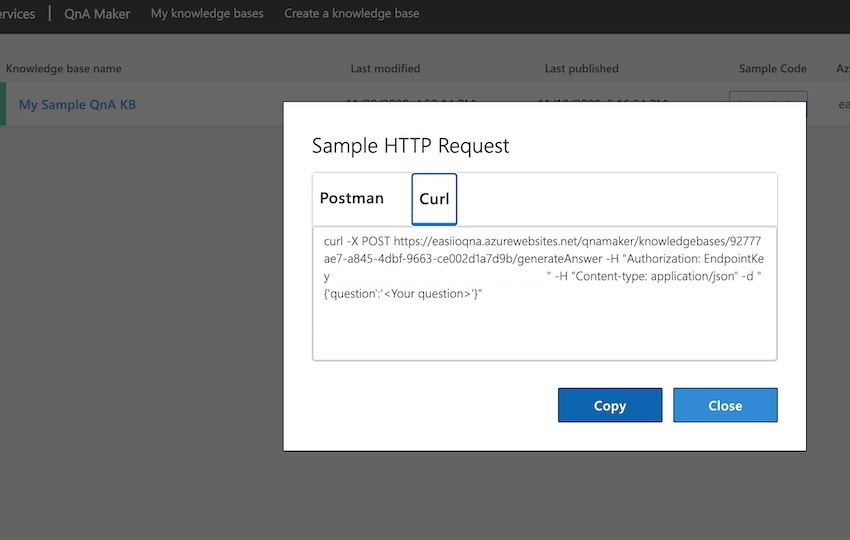
From the command line, run the curl command. You should get the answer from the Microsoft QnAMaker service.
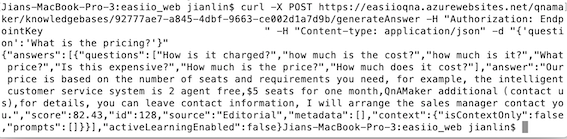
With that, it means our QnAMaker working from HTTP POST curl request. Now we are ready for the next step, to create the node.js service to bridge the Easiio webchat with QnAMaker.
Create Node.js code for Microsoft QnAMaker
To convert the Microsoft QnAMaker API return message format to the format that works for Easiio webchat, we implement the simple Node.js service to do the conversion. It takes HTTP POST requests from Easiio webchat and sends the request to the Microsoft QnAMaker. After the QnAMaker response, it will extract the response text and send it to Easiio in the appropriate format. See the code sample below.
//////////////////////////////////////////////////////////////////
// Easiio, Inc, Call Center https://www.easiio.com
// Sample code to send email. This could be use with Easiio
// IITR with ms qnamaker_chatbot API.
//
///////////////////////////////////////////////////////////////////
const http = require('http');
var url = require( "url" );
var queryString = require( "querystring" );
var sess = {};
"use strict";
var formOutput = '<html><body>'
+ '<h1>Zendesk support ticket example</h1>'
+ 'Please use post for the API request.</body></html>';
const server = http.createServer(function (request, response) {
try {
if(request.method === "GET") {
console.log ("Get request url ." + request.url );
} else if(request.method === "POST") {
if (request.url === "/azure_qnamaker_chatbot") {
var requestBody = '';
console.log("post inbound 1.0");
request.on('data', function(data) {
requestBody += data;
if(requestBody.length > 1e7) {
response.writeHead(413, 'Request Entity Too Large', {'Content-Type': 'text/html'});
response.end('<!doctype html><html><head><title>413</title></head><body>413: Request Entity Too Large</body></html>');
}
});
request.on('end', function() {
console.log("post end", requestBody);
var formData = JSON.parse( requestBody );
var request1 = require("request");
if (formData == null || formData.uuid == null || formData.text == null) {
response.write('[ { "id": 1, "action": "play", "text": "missing to, from, uuid or text" }]');
response.end('');
return;
}
console.log("send qnamaker", formData.uuid , " ", formData.text);
var options ={
url: 'https://easiioqna.azurewebsites.net/qnamaker/knowledgebases/your_urlkey/generateAnswer',
method:'POST',
headers:{
'Authorization': 'EndpointKey your_endpoint_key',
'Content-Type':'application/json'
},
body: JSON.stringify({'question': formData.text })
};
request1(options, function (error, response1, body) {
if (error) throw new Error(error);
console.log("send azure message", formData.uuid , " ", response1, " ", body);
var responseJson = JSON.parse (body);
if (responseJson != null && responseJson.answers != null)
console.log("send azure message 1.0");
if (responseJson.answers[0] != null)
console.log("send azure message 1.1");
if (responseJson != null && responseJson.answers != null && responseJson.answers[0] != null && responseJson.answers[0].answer != null)
response.write('[ { "id": 1, "action": "play", "text": "' + responseJson.answers[0].answer + '" }]');
else
response.write('[ { "id": 1, "action": "play", "text": "incorrect response." }]');
response.end('');
});
});
}
} else {
response.writeHead(405, 'Method Not Supported', {'Content-Type': 'text/html'});
return response.end('<!doctype html><html><head><title>405</title></head><body>405: Method Not Supported</body></html>');
}
} catch (err){
console.log(err);
}
});
const port = process.env.PORT || 1340;
server.listen(port);
console.log("Server running at http://localhost:%d", port);
Make sure to change the url to your service URL and replace the Authorization endpoint key with yours. You can find it from the following Service Settings console page.
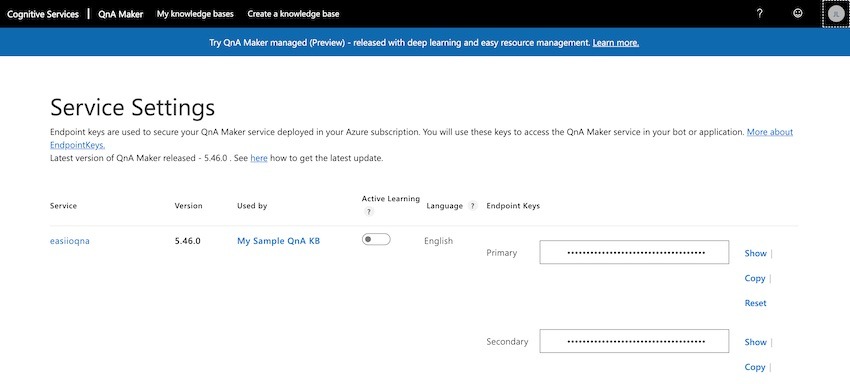
Run the service on your node.js environment using the command "node ms_qnamaker_chatbot.js". Use the following curl command to test your node.js service.
curl -H "Content-Type:application/json" -X POST -d '{"uuid":"contact@easiio.com","text":"what is the pricing?"}' http://localhost:1340/azure_qnamaker_chatbot
response:
{"answers":[{"questions":["How is it charged?","how much is the cost?","how much is it?","What price?","Is this expensive?","How much is the price?","How much does it cost?"],"answer":"Our price is based on the number of seats and requirements you need, for example, the intelligent customer service system is 2 agent free,$5 seats for one month,QnAMaker additional(contact us),for details, you can leave contact information, I will arrange the sales manager contact you.","score":82.43,"id":128,"source":"Editorial","metadata":[],"context":{"isContextOnly":false,"prompts":[]}}],"activeLearningEnabled":false}
send azure message 1.0
send azure message 1.1
With the response from the node.js code, we are sure that the node.js and Microsoft QnAMaker is now working together. We can now start step 3. That is to integrate the Easiio Website chat with the Microsoft QnAMaker.
Integration of Easiio Webchat
Last step, we need to use the node.js API to allow Easiio ITR bot API to interact with Microsoft QnAMaker.
First, you need to signup an Easiio account, which is free for the website chat feature. Please use the signup link to signup.
Second, configure a Visual ITR using the Advanced Configuration->Visual IVR menu to create a new ITR (Interactive Text Response). See the ITR instruction for how to create ITR. We use the ITR already created and add a Curl (HTTP API call) element. Curl is an API call to an external HTTPS API, the response from the API will be play directly to the chat windows as a response by the QnAMaker. As you can see, we set the curl command to use Command index 0, which means the default chat will go to the QnAMaker. Unless specifically asked for a live agent.
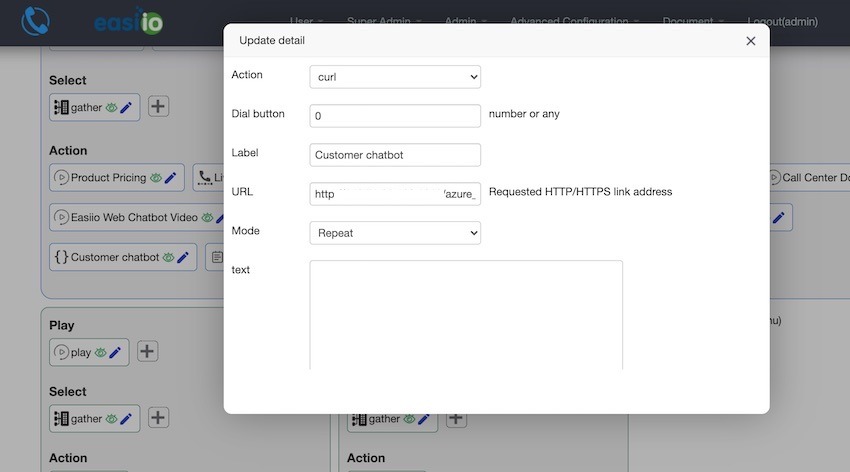
After it is configured on the website, we can now test it. You can test it using easiio.com website. Just type hi to start chatting with the Microsoft QnAMaker. For example, You can ask a question such as "What is the pricing?", "Do you support IVR?" etc. As the following screenshot.
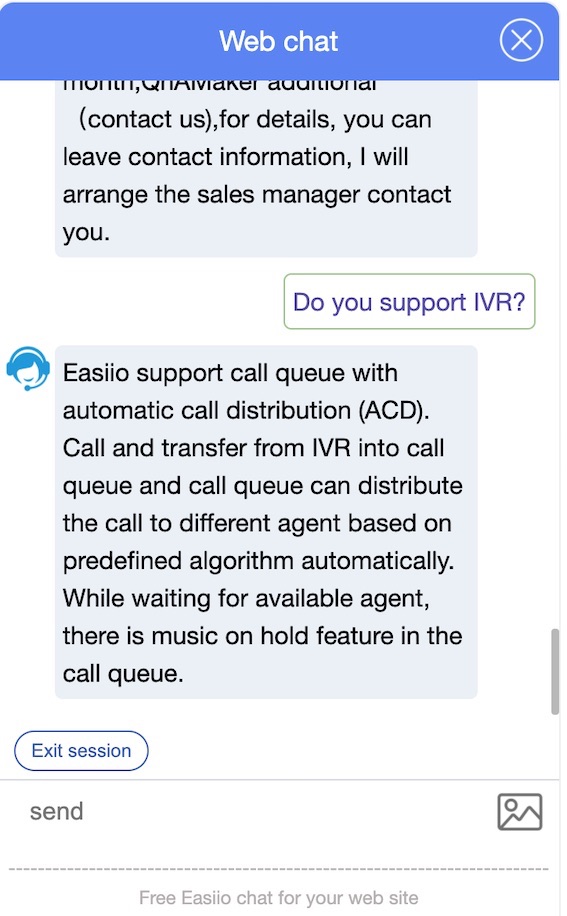
Conclusion
In this blog, we show how to integrate Microsoft QnAMaker with Easiio website chatbot/live chat plugin using a Node.js program as a bridge. It works nicely with relatively easy configuration steps. If you already have the service configured, it is very easy to use Easiio website chatbot/live chat platform to allow your website visitor to use the service. That means you can build one chatbot and use it with your website. On the other hand, Easiio website chatbot and live chat also support live chat with agents with many distribution algorithms and many advanced contact center features. Signup for free and experience the power of the Easiio website chatbot/live chat tool. It can help to make your website's customer service and sales a very successful operation.
In addition, Easiio also provides powerful call center software and business phone systems. Please check the website and signup.